Week 2
Assignment 01
Conway’s Game of Life
Instructions
Your assignment is to build your version of the Game of Life using openFrameworks. This will give you an opportunity to play with an image’s pixel data.
- Use an
ofImage
as your 2D canvas. - Set black
0
as the pixel value for a dead cell, and white255
as the pixel value for a live cell. - Set the initial values to whatever you want. You can use random values or try a pattern.
- Neighbors are the pixels on the top-left, top-center, top-right, middle-left, middle-right, bottom-left, bottom-center, and bottom-right (8 neighbors total).
- Make sure to handle edge cases appropriately! You can either ignore the invalid neighbors or wrap around the texture.
We will follow the same rules as the original game of life:
1. Isolation: a live ⬜ cell with less than
2
live neighbors will die 😥.2. Overcrowding: a live ⬜ cell with
4
or more neighbors will die 😵.3.Reproduction: a dead ⬛ cell with exactly
3
live neighbors will live 🐣.Results!
Version 1: start from the random value
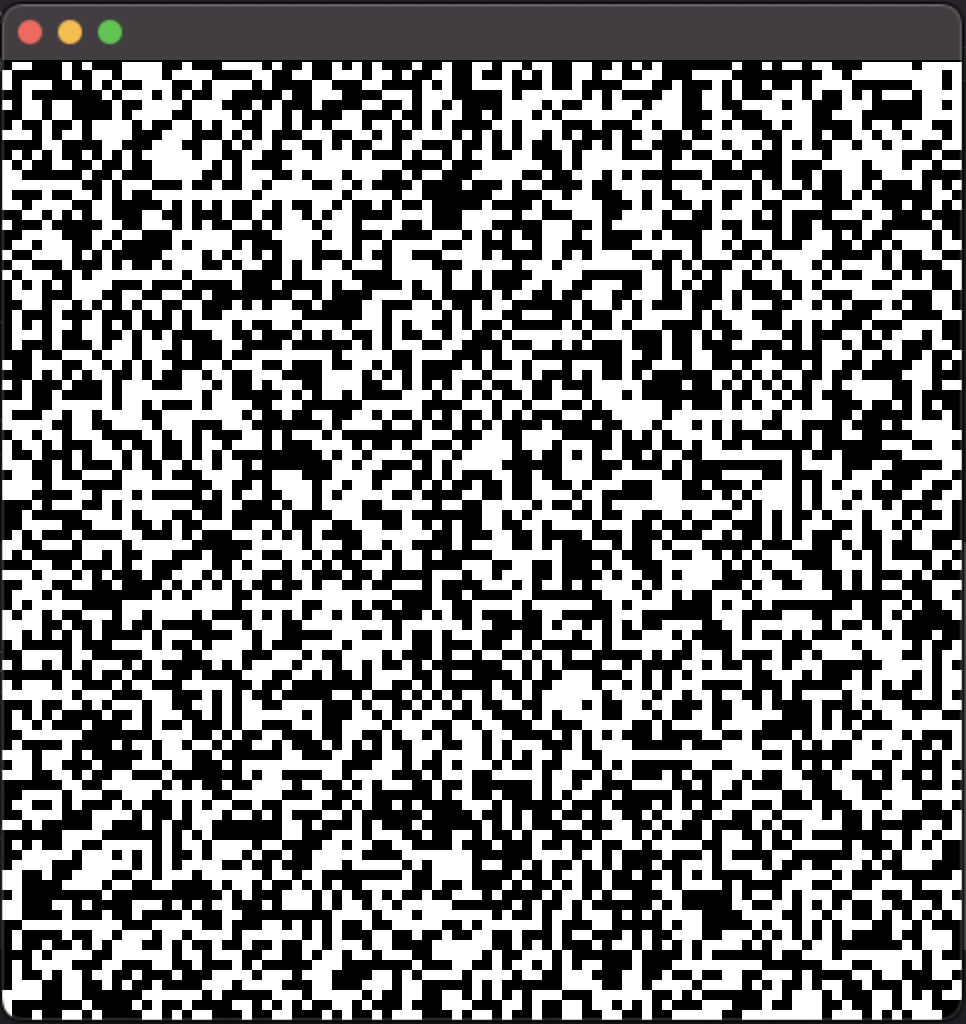
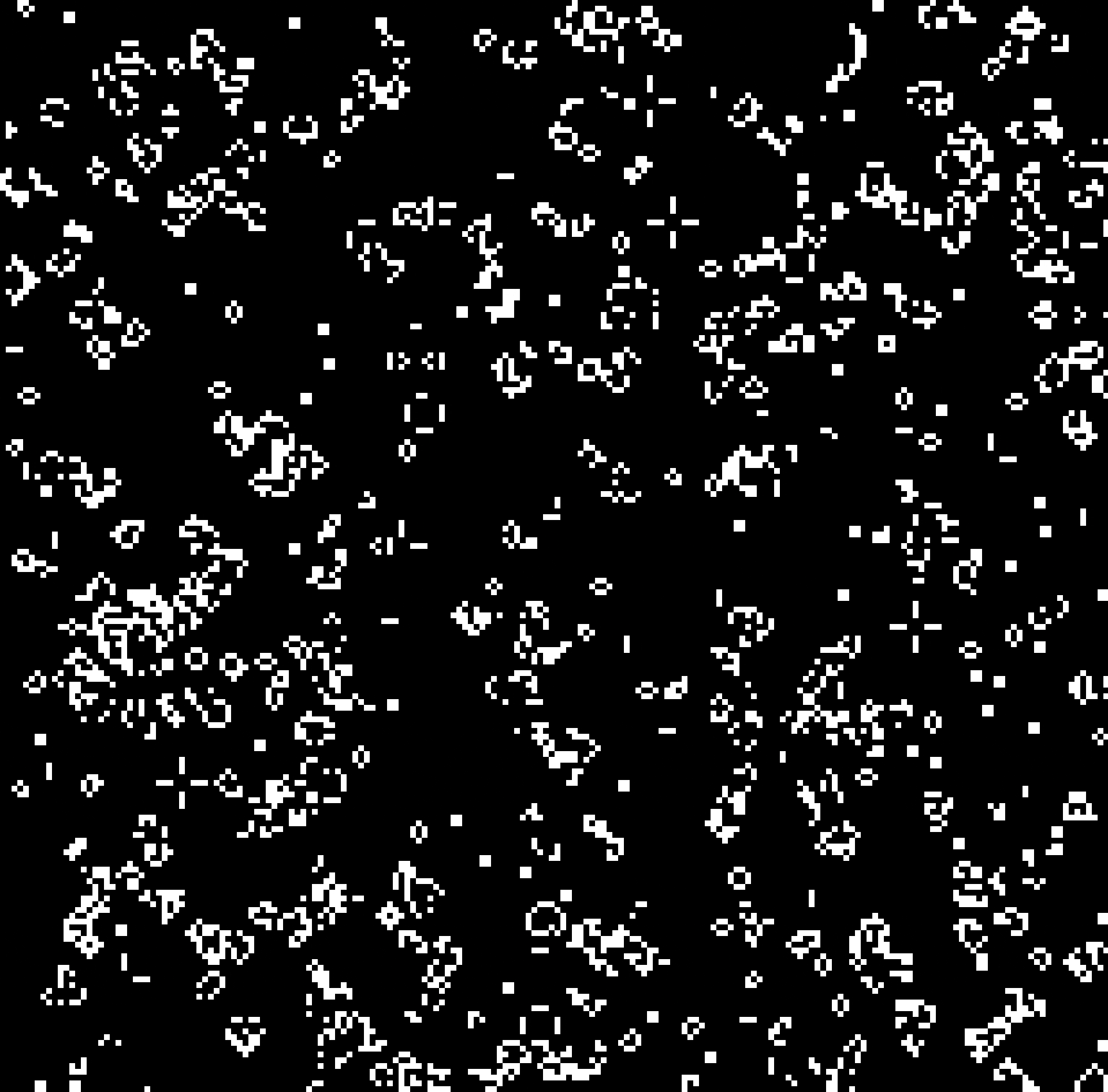
Version 2: start from an image
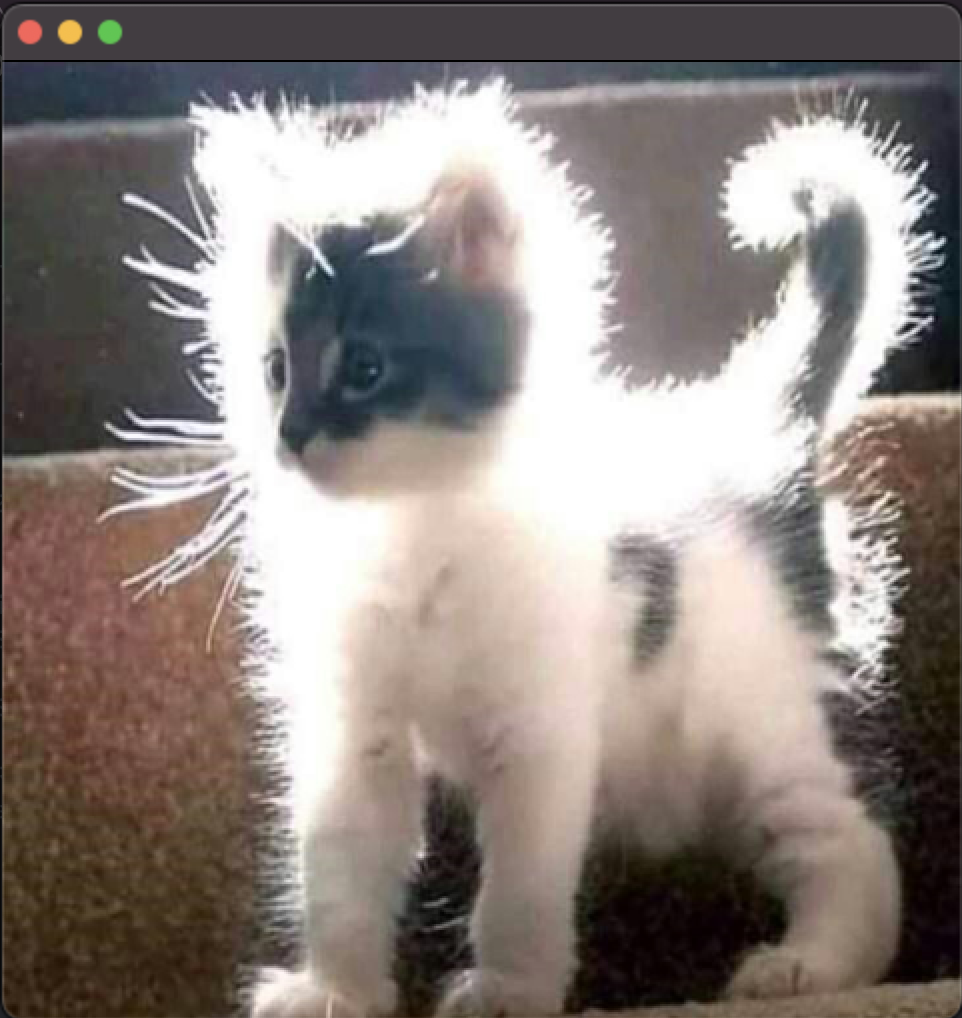
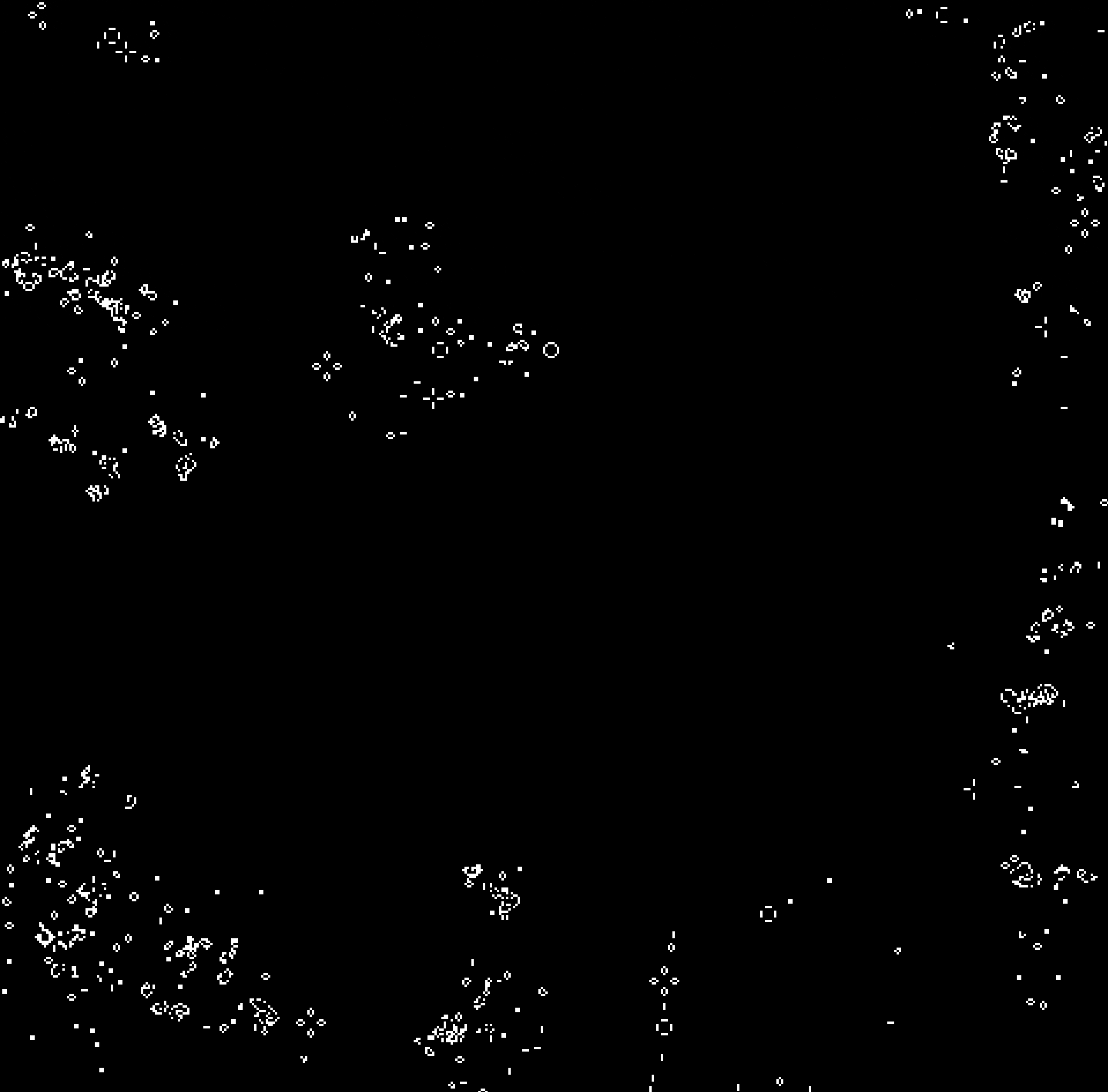
Version 2: start from an image
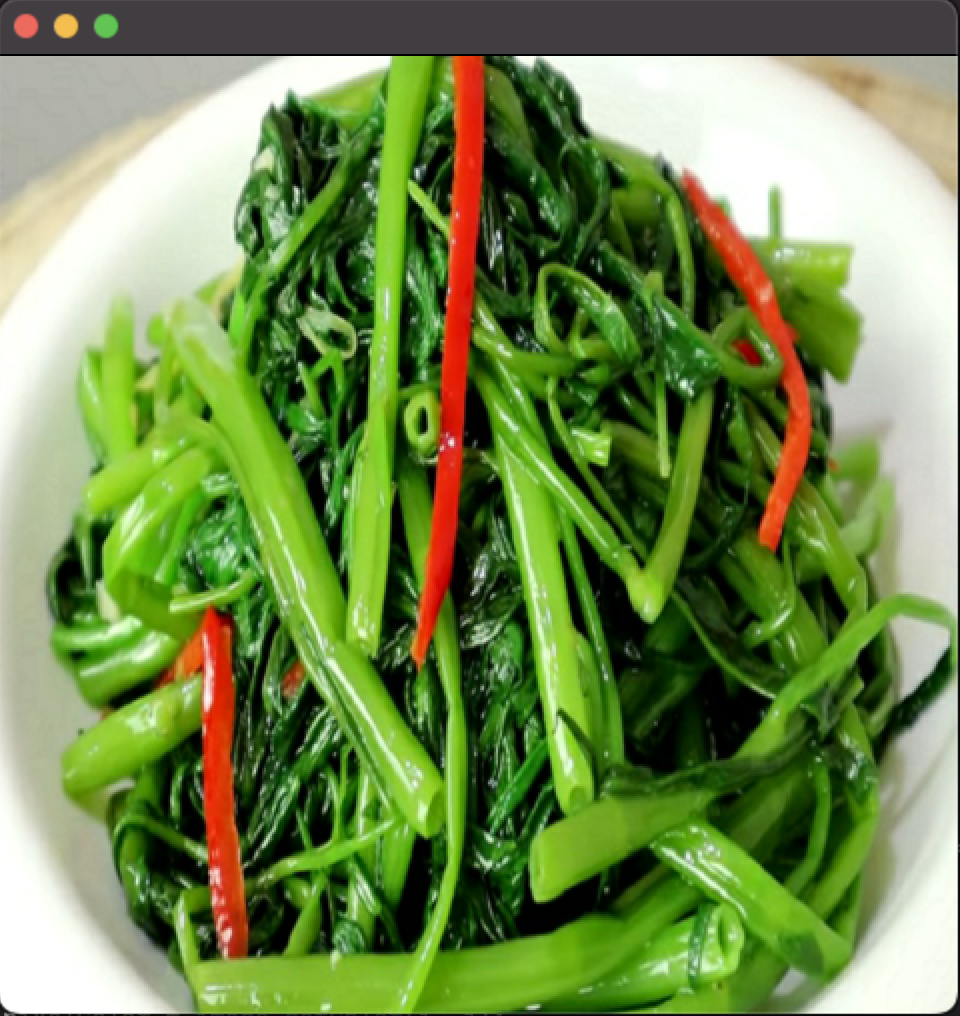
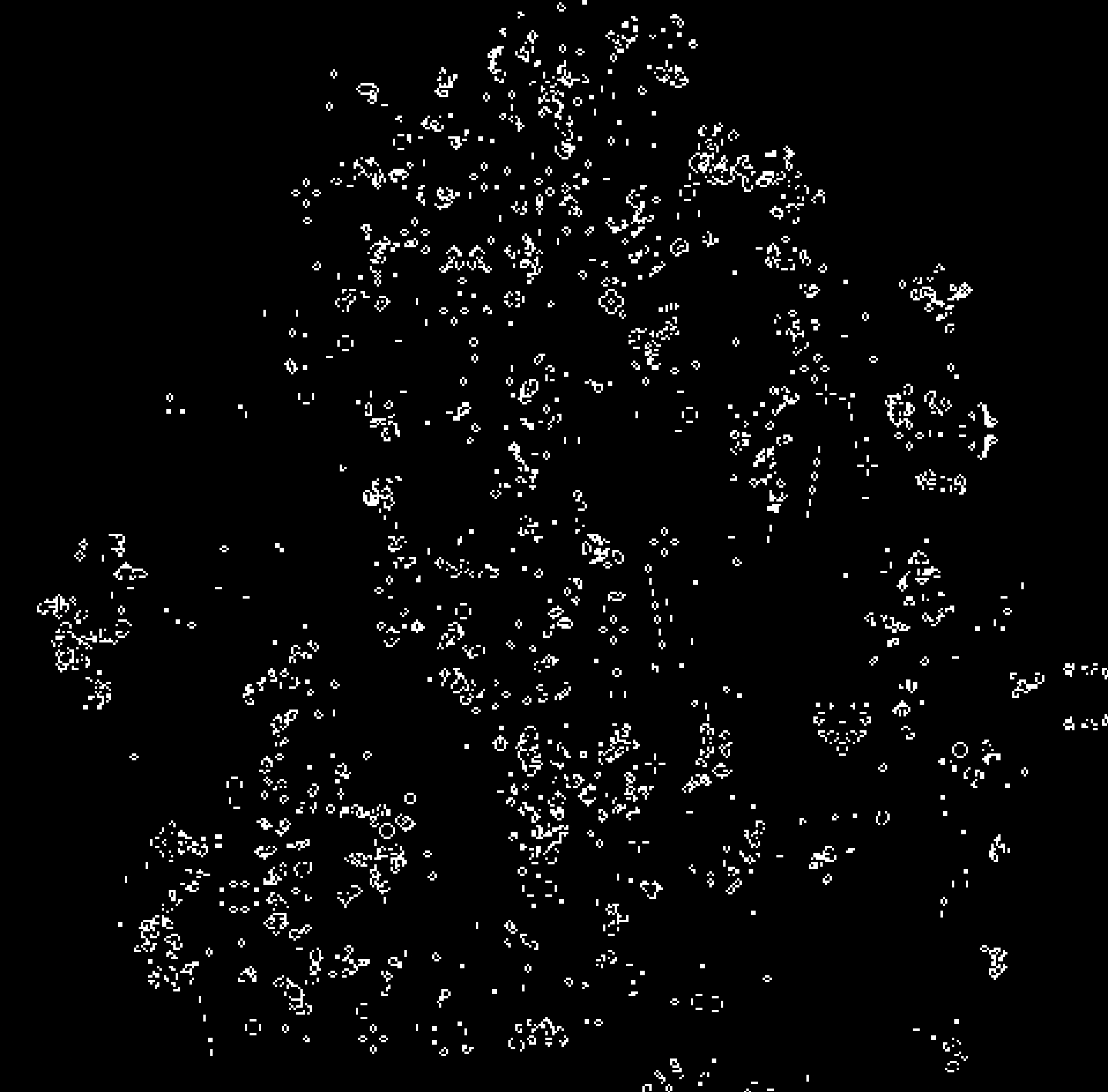
Code!
Before code in the editor...
I thought that I needed a function to check the neighbors and how to create it by for loop.
Also, how to define the first moment, the process of keeping the following moment data, and then draw them out.

Details
❶ Global Part
Define a function to count how many neighbors are true!
Also, I found the "int variable" needs "const" to make it a fixed-length array!
❷ Setup
I did the first version with the random function to decide every pixel's value( = 0 or 1).
Also, I did another version to get the image and resize it to the window size. After that, check every pixel's RGB value to decide whether it will be 0 or 1. Because of the image, I can get the same starting point.
❸ Update
Calculate the next generation based on the rules, then copy the next generation to the current grid!
❹Draw
Draw the rectangles!
Draw them on the position( i*cellsize, j* cellsize) can make the bigger one, but it will make it bigger than the default window size. ( but adjust the window can see the other parts!
==== [update!]====
Bonus Points!
If you are looking for an additional challenge, try adding the following extra features:
- Press a key to pause / start the simulation.
- Press a key to reset the grid to random values.
- Press a key to set the grid to all live cells, and another for all dead cells.
- Click the mouse on a cell and toggle its state!
Add
“p” for pause ,
“s” for restart and
“r” for random
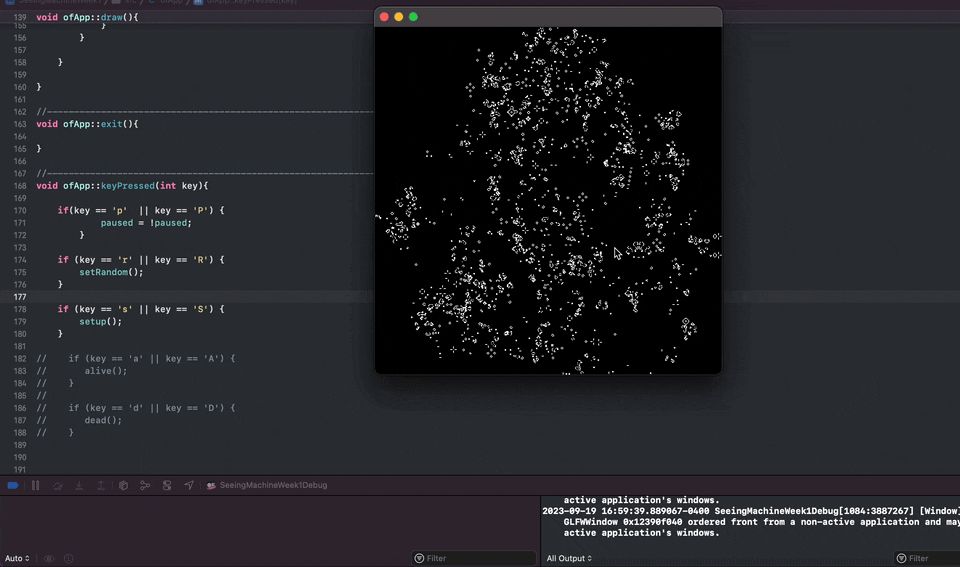
-
How do I control the cell size if I want to play with it? (Because I define it as a global variable, which can not be a variable length array.
- I tried to set the pixel to alive and dead, but if all of them are set to the same status, they will all be dead in the next moment.